I have been working on a project at the Mobile Robotics Lab of IISc (Indian Institute of Science), Bangalore, in which I have to design a vision based obstacle avoidance algorithm for robots. What does that mean? Well, I basically have to design a robot that uses nothing but a small camera to identify obstacles in its path. Since image processing and computer vision stuff is usually quite CPU intensive, it is difficult to implement this code on a small robot. Small microcontrollers can't handle that stuff. So, the solution I came up with involves a wireless camera that transmits video to a nearby "big" computer. This big computer runs all the dirty computer vision codes, identifies the obstacles, and then somehow tells the robot how to avoid them. This is where wireless communication comes in.
To create a wireless link, you could rip the guts of a cheap RC car and use its transmitter and receiver to control your robot. That's one technique. I did something similar a few years ago . This time, I wanted to do things a little more elegantly, without destroying an RC car. I found this really inexpensive RF transmitter/receiver pair at a local electronics shop in Bangalore:
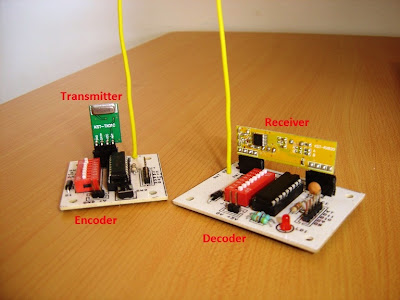
They cost only 200 INR (about 4 USD)...both transmitter and receiver. What a steal!
You can also find these online at Sparkfun:
Transmitter - http://www.sparkfun.com/products/8945
Receiver - http://www.sparkfun.com/products/8948
They cost a little more on Sparkfun, but they're still inexpensive. The ones on Sparkfun operate at 315 Mhz, but the ones I have, operate at 434 Mhz (like this one). I don't think that would make any difference in how you connect them.
To use these cheap RF modules, you could either connect them directly to your microcontroller/computer, or connect them with the help of parallel - serial encoder/decoder ICs.
I used the HT12E (parallel to serial encoder) and the HT12D (serial to parallel decoder) ICs. The HT12E is a 12 bit parallel to serial encoder. Of those 12 bits, 8 bits are the address code, and the remaining 4 bits are data. To send a signal, the address bits on the transmitter and receiver should be the same. It's like a password. You can use a single RF transmitter to control different RF receivers (at the same frequency) by configuring the address bits appropriately. All receivers would have to be set to different addresses. With 8 bits, you can create a total of 256 combinations.
Here's what the serial data coming out of the encoder looks like, in an oscilloscope:
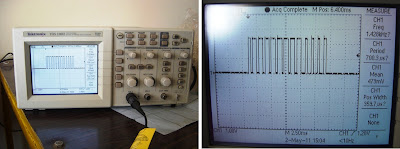
If you look closely, you'll notice that there are 13 peaks (instead of 12). I'm not sure what the first bit is for. (Probably a parity bit?) The 8 bits coming after the first bit make the address, and the last four make the data being sent. You connect your microcontroller's (or computer's) parallel output to the 4 input channels on the encoder. With 4 bits, you can create 16 unique commands. That is enough for my purpose.
For more on how to use these ICs with the Tx/Rx, check out this excellent article - http://www.botskool.com/tutorials/electronics/general-electronics/building-rf-remote-control
Here's a flowchart of my system:
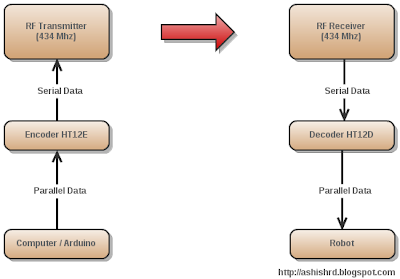
I've connected an Arduino to my computer which acts as an interface between the encoder and my computer. Here's the Arduino that I'm using:
It's a ModernDevice BBB Arduino Clone that I received from my friend Tom Boyd. I've been hooked to it ever since I got it! You should definitely get an Arduino if you don't already have one. It's the perfect tool for the programmer who enjoys playing with electronics. And it's also very easy and fun to use!
Tom has some excellent tutorials on electronics and programming on his website that keeps inspiring me! Check out some of his awesome Arduino projects - http://sheepdogguidecools.com/arduino/ahttoc.htm.
The robot I am using is a Microbric Viper robot that I received from Microbric a few years ago. The Microbric Viper comes with an IR module which can be used to communicate with a computer wirelessly. Although I've used it in the past, it's kind of difficult to set up because infrared communication requires line-of-sight. Moreover, the range is quite limited.
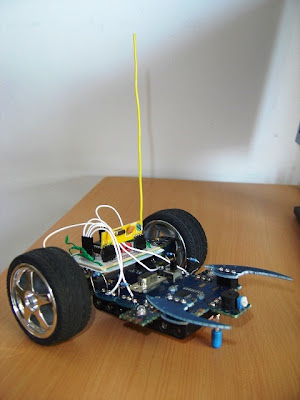
I'm not going to get into the details of connecting the encoders/decoders with the RF modules because it is already covered in detail in the article I mentioned earlier. I am however, going to share some difficulties I have faced in using these RF modules.
When I was testing the receiver on a breadboard, I was powering it with an AC to DC adapter. The voltage was fine, but the decoder did not work at all. Why? When I was debugging the circuit with an oscilloscope, I realized that the signal at the encoder side was fine, with 13 distinct peaks. However, when I checked the data at the receiver side, I realized that there was some sort of noise, and the peaks were not distinct. The address bits were too close together and sometimes even merging together. Since the address bits on the decoder side did not match with this noisy data, it rejected it. I figured that this noise could be caused by radio interference noise from the AC to DC adapter. So, I removed it and powered each of them (Tx and Rx) with two AA batteries.
The other thing I noticed is that if you connect the third pin of the RF receiver (which is either marked as "Data" or "CE") to the data-IN pin of the decoder , the circuit won't work. Leave it unconnected.
I'm using a 1/4 wave monopole antenna (6.8 inches) with the RF modules. It's just a single core wire. The range I get is amazing. I think I get about 100-120 ft (through walls), and 1000+ ft outside (line-of-sight)! More than enough for my purpose. When I test it outside, it just keeps working no matter how far I go. So, I don't really know its limit yet!
The Arduino communicates with my computer through a USB to Serial cable. If I want to make the robot move forward, I would send the character '1' to the Arduino. It would recognize this as a command and forward it to the transmitter. I can send four commands to the Arduino - '1', '2', '3' and '4'. On receiving these characters, it sets the appropriate data bits on the encoder and transmits the signal.
Arduino code:
On Windows, I use a C# application to send these commands to the Arduino. On Linux, I just use the terminal. My Arduino shows up as /dev/ttyUSB0. To send the command '1', I write...
echo -n "1" > /dev/ttyUSB0
This makes the robot move forward. For a more interactive session, you can use the screen command:
screen /dev/ttyUSB0 9600
More on this here - http://www.arduino.cc/playground/Interfacing/LinuxTTY
And finally, the thing you were probably waiting for...a video! -
I hope you enjoyed this post. My next step would be to put a wireless camera on this robot and test my obstacle avoidance algorithm. Wish me luck.
I hope the information I've shared helps you build your own radio controlled electronic devices and robots!
So what did you think? I'd love to hear your feedback in the section below.
Ashish
136 comments:
this is some really good stuff. i look forward to your posts and they never fail to impress. thanks for sharing
Good job done Ashish, all the best for your future projects.
Anil Mishra
Nice post, those transmitters/recievers look interesting, I'll have to pick some up someday!
Nice project. I put together a little proof of concept last week...I was driving a 4x20 LCD screen utilizing my Arduino and Linux box. Our solutions are very similar...I was doing an "echo -n [message] > /dev/ttyUSB0" to push text...pretty slick.
Take a look if you would like
http://swantron.com/command-line-lcd-arduino-interface/
Cheers
Nice!
Lucky that you are in bangalore, we have no shops like this in delhi.
Would it be possible for you to ship the Rx/Tx modules to delhi?
My email is sarangthestig(@) gmail.com
Thanks@
Nice!
Lucky that you are in bangalore, we have no shops like this in delhi.
Would it be possible for you to ship the Rx/Tx modules to delhi?
My email is sarangthestig(@) gmail.com
Thanks@
@Swantron: Cool project! Our solutions are similar. You gotta love Linux!
@Anonymous: Yes, there are shops in Delhi. Go to Lajpat Rai market (near Red Fort)
hi ashish...
could you please post the pcb layouts of RF transmitter and reciever! thanx
This is really awesome and helpful to many others.Thanks for making this available to everyone.Waiting for your future projects.
@Aficionado: The transmitter/receiver PCB was available as a kit, and I don't have its layout.
You can create your own layout based on the circuit on this page - http://www.botskool.com/tutorials/electronics/general-electronics/building-rf-remote-control
you're projects are just amazing and innovative. sure would like to learn some stuff from you. even i stay in bangalore.....where do you stay(may be we can meet up some time). and where in bangalore do you get all these stuff?(S.P. road i presume)
Are you a school student or an engg. student?
Great work Ashish. Best wishes.
Keep doing good work dude.
Thank you. was looking for this. will check it now by applying it.
Hi man!
Congrats for this work, it's really good!
But I have one question: I tried once do this (serial wireless communication) using a Atmel microcontroller and its serial comunication module (without encoder/decoder) and it didn't work!
Theoretically, it should work, huh?
Do you know the difference between a UART and these encoders?
Did you try do that?
Thanks a lot!
@franklin: Sorry for taking so long to respond! I had not checked this post for a long time. Anyhow, you said:
"I tried once do this (serial wireless communication) using a Atmel microcontroller and its serial comunication module (without encoder/decoder) and it didn't work!"
I think it should have worked. You don't have to use an encoder/decoder. The encoder is basically just translating the parallel input data to serial (for the transmitter).
Did you check the activity on the receiver side with an oscilloscope?
First of all, great post! I had not realised the first 8 bits were the address and the final 4 were data bits. Makes perfect sense now. I noticed that there were 13 peaks while reading the encoders output on an oscilloscope too. My thought is that perhaps the first peak is a wake up call for the receiver.
Thanks for the tutorial.
Nahdoa
Hi Ashish,
its a nice project. By the way your blog posts are great.
I would like to buy the Tx/Rx module and those encode/decoder circuits for one of my projects.Can you tell me where did you buy those in Bangalore? SP road i guess.
If so is there any specific model name you used to buy them?
Thanks for your reply in advance.
So have succeeded in it?
hi ashish!!i'm a big fan of your projects.thanks for posting so many projects and making them so easy!
@cartier: Yes! The obstacle avoidance robot is working nicely...I'll write a blog post on that soon. Too much work right now. :P
@elamaraon: Check Om Electronics on SP road.
i want to know how to use wireless camera in a mobile robot controlled by computer i read ur article it was awesome plz help me and if u have any program regarding above topic.
if u have that program plz share it in ur blog or just mail me
my email id is rmmechrocks07@gmail.com
i'll be highly obliged
I have been looking for a wireless camera but the ones I have found online are quite expensive.
Could you suggest some place I could get one?
hey anyway i can get contact with you? please email me at stormrobot@live.com.
Thanks
Thanks for the info Ashish.
Hey Ashish,
Great work here. Do you have an email I can grab you on I have a few questions to ask you about your builds.
Thanks
Ryan
Gud work. i'm also doing a similar work as my last year project . It is wireless PC controlled robotic arm. your blog is quite intresting continue your work :)
hi ASHISH!
Do you know what is the output voltage of the receiver?
thanks
Wow, it is really fantastic. I have used it. It is looking so cute. Love your blog.Best wishes for you future blogging career. Thanks
I am amazed to see such an amazing post. Keep up the good work.
Nice information, many thanks to the author. It is incomprehensible to me now, but in general,
The usefulness and significance is overwhelming.
Thanks again and good luck!
Nice post love your blog.This blog is awesome full of use full information that i was in dire need of. Thanks for this post. Keep it up.
Hello,
Very nice post. I like your blogging techniques and have bookmarked this blog as found it very informative. Keep it up.
Nice information, many thanks to the author. It is incomprehensible to me now, but in general,
The usefulness and significance is overwhelming.
Thanks again and good luck!
I am feeling very fresh and comfortable after reading you article. Nice work and keep doing well.
Very informative and impressive article. unique information.
Hello,
Very nice post, I like your blogging techniques and have bookmarked this blog as found it very informative. Keep it up.
hi
ur bot seems gr8.....
is it completly autonomous?
i mean if it sees an obstacle does it change its path on its own?
Useful content and awesome design you got here! I want to thank you for sharing your solutions and taking the time into the stuff you publish! Sublime work!e
very cool dude!!!!!!!
Thanks for the awesome post. Keep on going; I will keep on eye on it.
I am amazed to see such an amazing post. Keep up the good work.
Hello,
Very nice post, I like your blogging techniques and have bookmarked this blog as found it very informative. Keep it up.
This is one of my favorite i like this very much this is one of my favorite keep it up........ well done...
is there a way to send more than 4 commands?
Nice post love your blog.This blog is awesome full of use full information that i was in dire need of. Thanks for this post. Keep it up.
Very nice post. I like your blogging techniques and have bookmarked this blog as found it very informative. Keep it up.
Thank you for such a wonderful post.
I enjoyed every bit of it.
Hello,
Very nice post, I like your blogging techniques and have bookmarked this blog as found it very informative. Keep it up.
Nice post.and it is Amazing post as well . :)
Thanks for the awesome post. Keep on going; I will keep on eye on it.
It’s a Good blog and congrats on having good Information, Keep it up! Thanks for sharing your knowledge with us!
Hi,
Nice post! Your content is very valuable to me and just make it as my reference. Keep blogging with new post! Unique and useful to follower.
If it would have been split into manageable sections covering each technology rather than cramming it all into one, it would have been much better + easier to understand.
can u please explain the coding
"PORTD = B00000100;"
wat it means
It’s a Good blog and congrats on having good Information, Keep it up! Thanks for sharing your knowledge with us!
Really nice blog, very informative. Thanks dude for wonderful posting. Keep it up in the future as well.
Hi,
That is fabulous that a wireless robot is now available.. and i thing more i would say that congrats on making this and congrats that it is working nicely....
Great Technology.
Very useful and informative content has been shared, I must say that your writing skills are very attractive. Moreover sharing information and acknowledging others is really very good job, keep it up.
Wow, it is really fantastic. I have used it. It is looking so cute. Love your blog.Best wishes for you future blogging career. Thanks
the concept i need for the school project communicating using pc
Good evening there, I am from Malaysia.
I would like to ask about the range for the car to move.
Is it possible to move around a house with just using transmitter and receiver?
Your reply is appreciated.
@AlbertTehP: It depends on how big your house is. The range I got was about 150 ft line of sight. It worked in my house without any problem.
will it work even if i use normal wires like the ones which i use to connect stuff on my breadboard as the antennas?? i've connected everything correctly as per my knowledge but doesnt seem to work.. pls help..
and will it work fine if i dont use any switches in the Tx? Just ground it for 0 and connect it to Vcc for +5 volts? This is what i am doing for all the pins D0 to D3 and the TE pin..
hi ashish i have a doubt that whether a wireless robot uses more power or wired one?????
good project
This website is providing the lot of topics are visible in this blog that to valuable info in this blog.
may I know the specification of this project? If I am going to use zigbee will it work? what is the connection of the arduino to pc? could you please send the schematic diagram of this project to may account?thank you..jrexsal@yahoo.com
Hey I'm looking forward to build this for our Electronic Project in College.. But I have a BIG Question... Where Can I buy this Vehicle Part with 2 Wheels, Motors and the Circuit ???
Can we use the same wireless process for laptops as it has an embedded keypad and also does it's parallel port can be connected with an encoder IC
and also how the commands of the keyboard travels to the parallel port..?
awesome work !!!!!!
can u plz explain the baseboard connections of rf tx&rx.....t link tt u have posted is not responding :(
plz do tis at t earliest.....
Hi Ashish, I would like to ask if you can design a video circuit for me. Please contact me for more details. Thanks & have a good day.
one doubt please.....u have mentioned that u have used viper robot kit in this project....we have to use Viper Robot Kit only or we can use any remote control car circuit.
hello Ashish..the info provided are very useful..could you please provide some info about electronic shop frm wer u bout RF transmitter/receiver and also does this RF transmitter/receiver come with encoder and decoder..Pls do reply..
Sir, I want to make an internet controlled robo via a laptop..... I've connected my robo to a laptop bt dont know how to connect the lapi to the the internet and share the data packets..... Please help me out......//
My Email Id-shashwat.krish.sriv@gmail.com
Sir, I want to make an internet controlled robo via a laptop..... I've connected my robo to a laptop bt dont know how to connect the lapi to the the internet and share the data packets..... Please help me out.
my emil xx11oo@windowslive.com
Hi, Nice post thanks for sharing. Would you please consider adding a shout out to my website on your next post? I will return the favor. Please email me back. Thanks!
Randy
randydavis387@gmail.com
Project provides very good information about interfacing adruino to computer.go on and on. all the best
Hey Ashish.
I'm a 11th standard student interested in designing a similar robot.
Please can you give me a list of all the components you've used?
I'm guessing you bought them from SP Road :P
here's my email id:
rahuldias69@gmail.com
really need your help! :)
this is one of my preferred tutorial from where I learn how to use the Ethernet shield. I add this tutorial to an article http://www.intorobotics.com/getting-started-with-arduino-ethernet-shield-tutorials-and-resources/ aiming to help beginners to understand how a robot can be controlled through internet
how ur controlling dc geared motar with out using L293D?
Hi. I really enjoyed my brief visit on your site and I’ll be sure to be back for more.
Can you please consider placing my website on your link list?
Please email me back.
Thanks!
Kevin
kevincollins1012 gmail.com
Please send me ur shop details to ramyashree2612 @gmail.com
I am interested your blog
RF Post processing
Great Article, You are Just Awesome have bookmarked this blog as found it very informative. Keep Writting!
hi ashish!!i'm a big fan of your projects.thanks for posting so many projects and making them so easy!
Really nice blog, very informative. Thanks dude for wonderful posting. Keep it up in the future as well.
Great Technology.Thanks for sharing....
Great Post,thanks for sharing this information looking forward for more posts
Duct inspection Robots | Duct cleaning Robots
your blog is recommended for every programmer specially which are learning Advanced Java Programming
Programming is combination of intelligent and creative work. Programmers can do anything with code. The entire Programming tutorials that you mention here on this blog are awesome. Beginners Heap also provides latest tutorials of Programming from beginning to advance level.
Be with us to learn programming in new and creative way.
Annual Maintenance Contract , IT Infrastructure Management Services , Servers, Storage Rental , AMC, FMS Services - Bangalore, Chennai, Hyderabad, Mumbai, Pune.
I have found this information to be extremely valuable which will definitely help others. Thanks for the article. Great!Enabled speakers in Bangalore | Projectors in Bangalore
Thank you for accepting comments about our site
شركة نظافة بالرياض
شركة رش مبيدات بالرياض
www.nileriyadh.com
شركة تسليك مجارى بالرياض
عندما تستعين بشركة متخصصة في عمل التنظيف الخاصة عندما تكون الشركة لها سمعة طيبة في مجال التنظيف ستحصل على منزل براق وأرضيات و حوائط نظيفة تماما مع الحفاظ على ألوانها من مواد التنظيف لنظافة شيئا ضروريا وخاصة عند تواجد اطفال صغار يخشي عليهم من الاتربة
شركة تنظيف واجهات بالرياض
شركة تنظيف مساجد بالرياض
شركة تنظيف بيوت شعر بالرياض
شركة تنظيف وصيانة مسابح بالرياض
صيانة سامسونج
صيانة ال جي
6 اكتوبر صيانة توشيبا
صيانة سيمنس 6 اكتوبر
6اكتوبر صيانة بوش
6 اكتوبر صيانة دايو
6 اكتوبر صيانة اريستون
6 اكتوبر صيانة وايت ويل
ديكور بالرياض
جبس بورد بالرياضشركة سحر اللمسات
Visa from pakistan
Zong 4G Free Unlimited Whatsapp
يمكنكم الأن صيانة كافة أنواع الأجهزة المنزلية مع توكيل كريازي و الذي يعتمد علي خبراء في مجال الصيانة العالمية تواصلو الأن
مع صيانة كريازي لطلب خدمات الدعم الفني المنزلي لصيانة الأعطال الكهربائية
استمتع الان بافضل عروض و خدمات الصيانات المتميزه لجميع الاجهزه الكهربائيه الان صيانة يونيون اير و علي اعلي مستوي الان من التاميز و بافضل ادقان الان من خلال توكيل يونيون اير افضل متخصصين الان تواصلو معنا الان
افضل مهندسين صيانات الان و علي اعلي مستوي الان من التميز الان من خلال صيانة كاريير افضل متخصصين صيانات الان و العمل الان علي اعلي مستوي الان من التميز من خلال افضل متخصصين تواصلو معنا توكيل كاريير الان من خلال موقعنا الان و احصل الان علي افضل الصيانات
تمتع الان بافضل صيانات من خلال بتوفير افضل متخصصين في مجالات الصيانات صيانة توشيبا حيث يتم الصيانة علي اعلي مستوي من الاحترافيه و الادقان و بافضل مستوي من الكفائه تواصلو معنا الان توكيل توشيبا من خلال موقعنا او من خلال خدمة العملاء الخاصه بنا و احصل علي افضل خدمات الصيانة
احصل الان علي افضل صيانة من خلال بتوفير افضل الصيانات صيانة سامسونج و من خلال افضل فريق عمل صيانة الاسكا لاعمال الصيانة بافضل كفائه و افضل خبره لنضمن لكم صيانات متميزه تواصلو صيانة الكتروستار معنا الان من خلال موقعنا و احصل علي افضل صيانة
اعمال صانات متميزه الان و علي اعلي مستوي الان وبافضل الاسعار و افضل الخصومات خدمة عملاء كلفينيتور الان وبافضل تركيبات الان احصل الان علي افضل صيانات الان و علي اعلي خدمة عملاء شارب مستوي الان من التميز الان من خلال خدمة عملاء كاريير متخصصين الان تواصلو معنا الان
يقدم صيانة بيكو افضل خدمات بيع كافة انواع قطع الغيار الاصلية من صيانة جولدي و بـ افضل الاسعار مع صيانة جنرال اليكتريك يمكنكم ايضا الحصول علي خدمات الصيانة الوقائية من صيانة جليم جاز تواصل معنا الان
مركز صيانة توشيبا بالبحيرة
Nice article, very nice article.
Thanks
ugc care approved list 2019
open access journals 2020 list
Thanks for sharing, nice post! Post really provice useful information!
Hương Lâm chuyên cung cấp máy photocopy và dịch vụ cho thuê máy photocopy giá rẻ, uy tín TP.HCM với dòng máy photocopy toshiba và dòng máy photocopy ricoh uy tín, giá rẻ.
Great Article & Thanks for sharing.
Oflox Is The Best Digital Marketing Company In Dehradun, Website Development company in Dehradun or Website Development Company In Saharanpur
http://alltopc.com/2020/08/31/portable-adobe-premiere-pro-cs6-free-download-portable-adobe-premiere-pro-cs6-free/
https://getdailybook.com/
Benjamin Briel Lee was very professional at all times, keeping me aware of everything that was happening, If I had any questions he was always available to answer. This was my first home purchase, I didn’t know much about the loan process, he made it very easy to understand the things I had questions about. I really enjoyed working with him.”
He's a loan officer working with a group of investor's who are willing to fund any project or loan you any amount with a very low interest.Contact Benjamin Briel Lee E-Mail: 247officedept@gmail.com Whats-App Number: +1-989-394-3740.
Very informative! dr trust weighing machine
Using your Microsoft Account associated with office to sign in will take you to the Dashboard for Office installation, If you are new to Office, you can create a New account and enter Product key to redeem and Install Office. office.com/setup
www.office.com/setup
श्री हनुमान चालीसा Hanuman Chalisa lyrics
श्री शनि चालीसा (Shri Shani Chalisa)
श्री शिव चालीसा (Shri Shiv Chalisa)
कंप्यूटर A-Z की शॉर्टकट Keys
Wow It's really nice information
raksha bandhan Movie
nice articel tecnology post is great
gyanpie
A ver Good Article for technology geeks.
Shri Gayatri Chalisa
Surya Chalisa
Shani Chalisa
تسليك مجاري المطبخ
شركات تسليك المجاري بالقطيف
USA pone Video
outsourcingall.com
USA SEX WEB carefulu.com
bd sex video rizik.com.bd
bd sex video ABEE85 CAPCUT
india sex video Spa in dhaka
bd sex video bestcallgirlservice.com
Good information
Union Bank Home Loan
personal loan kaise Le
Hey, You are doing great work. it is very useful to beginners.
graphic designing Training in Delhi
Latest News and Movie Watch Site atishmkv
Wow It's really nice information
Orkfriend
Checkout Library Pick Up Lines for Book Lovers in 2023
Want to download new hd movies!
United Kacche 2023 Web Series Download Filmyzilla
Want To Download Ajay Devgun's new Movie Bhoola in Hd
Bholaa 2023 Full Movie Download [480p 720p 1080p] | Ajay Devgan Bholaa Movie Download
Post a Comment